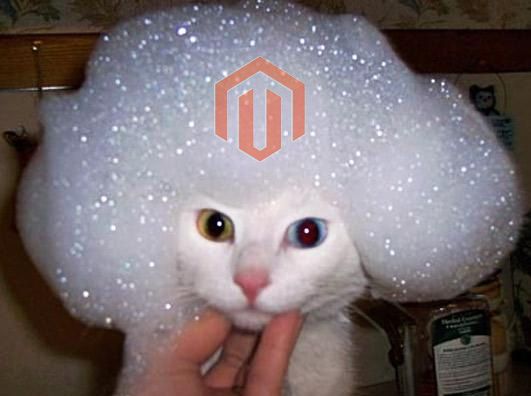
Are you a developer? Do you like Magento (at least 60% of the time)? Do you like SOAP APIs?
Is answering these questions as easy as a two year old saying yes, I am a kid. Yes, I like cookies. Yes, I want one now?
If so, read on. If not, I still really want to help you, so maybe contact us here and we can figure it out together.
This article is not intended to be a beefy description of what SOAP and APIs are. I’m assuming you already have some knowledge of the topic and we can jump in. What we are going to do is use Magento APIs to create a quote, assign addresses to the quote, add products to the quote, and then get a shipping rate for that quote.
Why? Consider you have a remote application that is managing orders and master inventory. That system periodically needs to pick up orders from Magento and check to make sure that the items in the order are still in-stock in the system. If they are not, maybe we want to cancel the item out.
As a result, we need a new shipping rate for the new order. In the native Magento functionality, we would need to cancel the entire order and create a new order. This workflow is a little clunky when we can just use Magento’s APIs to do a lot of this for us. Although I will not get into the process of actually canceling an individual item, I will show how to create a new quote with new products and get rates from it.
This is just an external script, but hopefully you can see how you can plugin your data and make this work for a variety of needs.
[php]
//
//
error_reporting(E_ALL);
ini_set(‘display_errors’, ‘1’);
include_once ‘app/Mage.php’;
umask(0);
$initializationCode = ‘admin’;
$initializationType = ‘store’;
Mage::app($initializationCode, $initializationType);
/**
* This is the path to the V2 wsdl of the magento api
**/
$wsdl = ‘http://yoursite.com/api/v2_soap/?wsdl=1’;
/**
* Create the soap client
**/
$client = new SoapClient($wsdl, array(‘trace’ => false));
$username = ‘mysoapuser’;
$password = ‘mysoapuserpassword’;
/**
* For every magento api session, we first need to login.
* The returned value is you API session_id which you need to
* Pass to every API call you make.
**/
$session_id = $client->login($username, $password);
/**
* The first thing we do is create the Magento shopping cart, or quote
*
* Here is detailed information and more examples from Magento:
* http://www.magentocommerce.com/api/soap/checkout/cart/cart.create.html
*
* We pass it the session_id from our login call and the store id we want
* create the quote for. The returned value will be the quote_id
* if it was successful, or nothing with no faults if it fails
*
**/
$cart = $client->shoppingCartCreate($session_id, ‘1’);
$addAddress = $client->shoppingCartCustomerAddresses(
$session_id,
$cart,
array(
array(
‘mode’ => ‘shipping’,
‘firstname’ => ‘Paul’,
‘lastname’ => ‘Briscoe’,
‘street’ => ‘117 N. 1st St.’,
‘city’ => ‘Ann Arbor’,
‘region’ => ‘MI’,
‘postcode’ => ‘48104’,
‘country_id’ => ‘US’,
‘telephone’ => ‘7345458017’,
‘is_default_shipping’ => 1
)
)
);
/**
* Just make sure to check that the quoteId was returned from the API
* Next we are going to start adding products into the new quote. The format is below.
* Keep in mind that you could easily loop through an array of order products.
*
* Note: getExternalOrder() does not actually exist, but if you have an order object
* or array of products you can use to get the info you need.
**/
$externalOrderProducts = getExternalOrder();
$products = array();
foreach($externalOrderProducts as $product){
$products[] = array(
‘product_id’ => $product->getId(),
‘sku’ => $product->getSku(),
‘qty’ => $product->getQty(),
‘options’ => null,
‘bundle_option’ => null,
‘bundle_option_qty’ => null,
‘links’ => null
)
}
/**
* Here make the API call back to Magento to add the product array to the quote
*
* For more information on this call see:
* http://www.magentocommerce.com/api/soap/checkout/cartProduct/cart_product.add.html
*
* Returns True if the product successfully added to the quote
**/
if($addAddress) {
$addProduct = $client->shoppingCartProductAdd(
$session_id,
$cart,
array($products)
);
} else {
echo "Address did not add.";
}
/**
* Again, check that the product added successfully and then simply call the Shipping List API
* For a list of available shipping methods for this quote.
*
* For more information on this call see:
* http://www.magentocommerce.com/api/soap/checkout/cartShipping/cart_shipping.list.html
*
**/
if($addProduct) {
$shipping = $client->shoppingCartShippingList($session_id, $cart);
} else {
echo "Product did not add.";
}
echo "<pre>";
var_dump($shipping);
echo "</pre>";
//
//
[/php]
That’s about it for this demonstration. I’m sure you can see how this could be helpful in some situations. If you have any comments, suggestions, or questions, please feel free to post!